Understanding URL Decode: The Essential Guide for Web Development
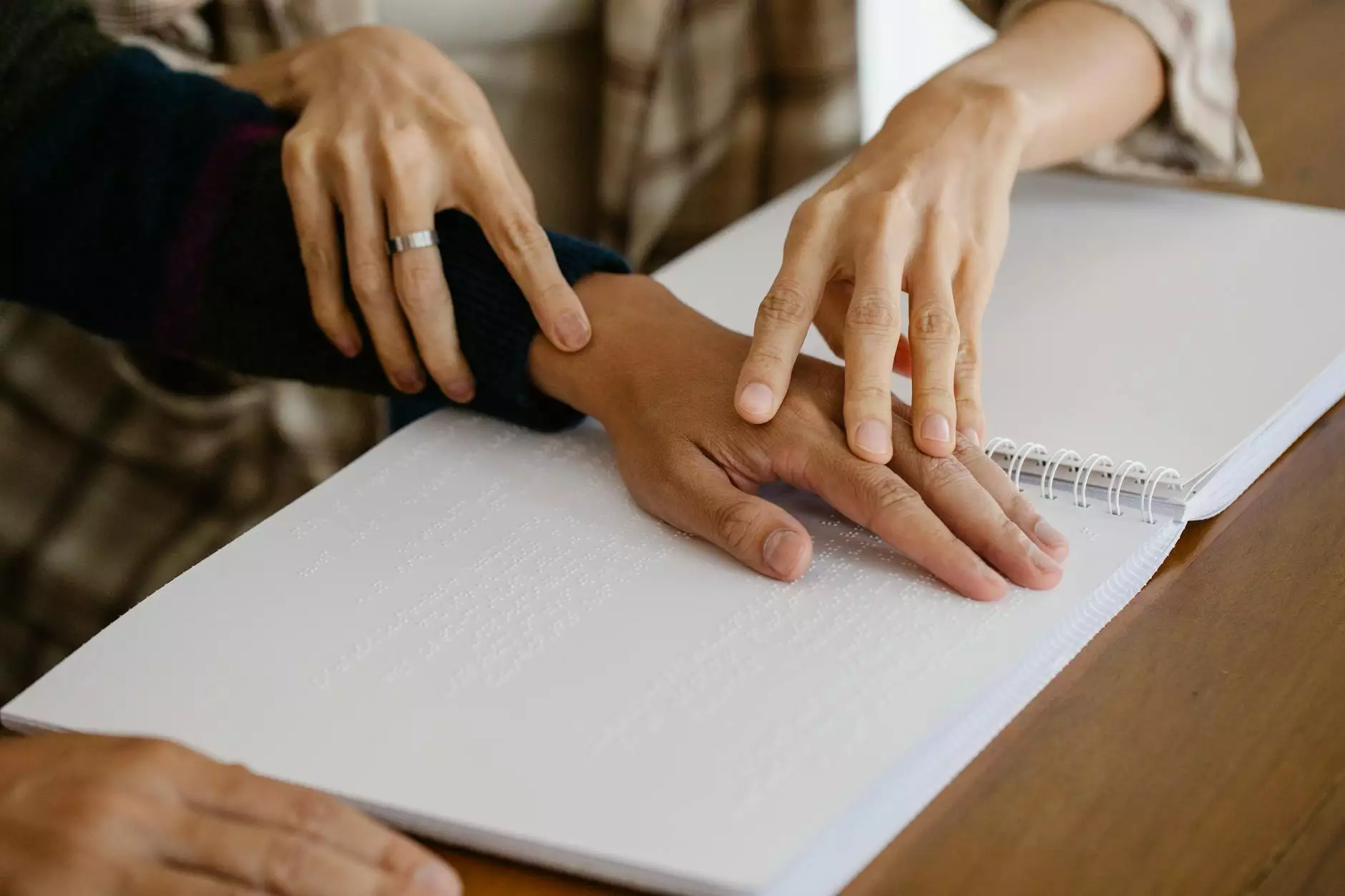
In today's digital landscape, effective web development is crucial for businesses aiming to stand out. One of the foundational concepts that supports this framework is the process of URL decode. Whether you are a seasoned developer or a novice just stepping into the world of programming, understanding URL decoding can enhance your projects significantly.
What is URL Decoding?
URL decoding refers to the process of converting a URL that has been encoded into a standard format. URLs are encoded to ensure they can be transmitted over the internet without errors. During this process, special characters are replaced with a percent sign (%) followed by two hexadecimal digits representing the character's ASCII value.
Why is URL Decoding Important?
- Improved Data Transmission: Encoded URLs allow for safe data transmission across different systems without corruption.
- Enhanced User Experience: Decoding ensures users can access URLs as intended, making navigation smoother.
- SEO Benefits: Proper handling of URLs can indirectly affect search engine rankings by preventing broken links and ensuring clean, readable URLs.
The Process of URL Decoding
The URL decoding process is executed through various programming languages, each offering its own built-in functions. Understanding how to leverage these functions is critical for effective web development. Let's explore some common implementations:
URL Decode in JavaScript
In JavaScript, the function decodeURIComponent() is widely used for decoding URLs. This function can convert encoded URI components back to their original format.
const encodedURL = "Hello%20World%21"; const decodedURL = decodeURIComponent(encodedURL); console.log(decodedURL); // Output: Hello World!URL Decode in Python
Python developers utilize urllib.parse.unquote() for URL decoding. This function decodes a query component of a URL, transforming it into a usable format.
import urllib.parse encoded_url = "Hello%20World%21" decoded_url = urllib.parse.unquote(encoded_url) print(decoded_url) # Output: Hello World!URL Decode in Java
For Java developers, the java.net.URLDecoder class is commonly utilized. Here's how it works:
import java.net.URLDecoder; import java.nio.charset.StandardCharsets; public class Main { public static void main(String[] args) { String encodedURL = "Hello%20World%21"; String decodedURL = URLDecoder.decode(encodedURL, StandardCharsets.UTF_8); System.out.println(decodedURL); // Output: Hello World! } }Common Use Cases for URL Decoding
Understanding the scenarios in which URL decoding is necessary can enhance your web development projects significantly. Here are several situations where decoding is essential:
- Web Forms: Form submissions may include encoded URLs in their action attribute, necessitating decoding for proper processing.
- API Requests: Many APIs return encoded URLs in their responses, requiring decoding to extract meaningful data.
- Search Queries: When users search for content, the queries often get encoded, and decoding is necessary to navigate accordingly.
Best Practices for URL Decoding
When working with URL decoding in your projects, keep the following best practices in mind to ensure optimal performance and security:
- Always Validate Input: Ensure that any URLs being decoded are from trusted sources to avoid injection attacks.
- Handle Exceptions: Implement error handling to manage cases where decoding fails due to malformed URLs.
- Maintain a Clean Code Structure: Organize your code effectively, especially when dealing with multiple programming languages.
Conclusion
In conclusion, URL decode stands as a crucial element within the web development sphere, enabling seamless communication, enhanced user experiences, and improved SEO practices. By mastering the fundamental principles and practical implementations of URL decoding across various programming languages, you position your projects for success. The digital landscape is ever-evolving, and staying ahead in the game requires a thorough understanding of these essential processes.
As you embark on new web development projects, let the knowledge of URL decoding guide your decisions and strategies. For further exploration and professional insights into web design and software development, be sure to connect with semalt.tools—your partner in crafting robust digital solutions.